In this short post we show you how to become a web3 developer fast. Set up your development environment and deploy your first smart contract. It's easy really. Just follow the steps and join our Discord if you have more questions.
What you need to become a web3 developer?
First of all, it's really not that hard. Keep that in mind from the beginning so you are not afraid to fully dive into it.
Secondly, you need to understand that web3 consists of multiple elements:
-
The Blockchain, which is run by Nodes or Validators aka. some people who want to run those and get rewarded for doing so. Smart Contract run on top of the blockchain. Aka. "the backend".
-
User Interfaces, which connect to smart contracts to make it easy for users to use their features. Aka. "the frontend".
-
Wallets, which acts as the user's identity and also function as asset storage tools. Well technically they don't store the assets, those are stored on the blockchain itself but via the Wallet the user can get access to their on-chain assets. More on that later :)
And that's it.
What does it mean for you as a web3 developer?
You need to run all of the 3 items mentioned above on your local machine in order to be able to code for web3 :)
You need a local Blockchain, a User Interface and a Wallet running locally.
And once your code runs as intended you need to deploy all of those 3 to "production".
The industry calls this "mainnet". But's it's production trust me 😜
If you have questions, make sure to join our Discord, where we are available for you: https://discord.com/invite/J32drKNJtK
Set up your development stack
There are two development frameworks you can use, Truffle or Hardhat that run a local blockchain and tools you'll need for development for you.
In this post we will use Truffle simply because it's still the most popular one and is easy to get started with. We have a ton of code snippets, tips & tricks with Truffle that you can profit from.
With that, let's get started 👾
Pre-requisites
You need to have npm & NodeJS installed before you can continue.
You need to open a Terminal window on your machine.
On Mac you can use Spotlight, search for "Terminal" for example and open it.
Red text like this
means "DO THIS".
So if you simply follow all the red text
you will not miss any steps you need to take.
The non-red text in between is for explanation and deeper understanding.
Install npm
The long explanation on how to get npm and NodeJS installed can be found here: https://docs.npmjs.com/downloading-and-installing-node-js-and-npm
The short answer is "run these commands one after another":curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
nvm install node
To double check if all went well, you can run these commands to check the version of npm and NodeJS. If you get the versions printed in the Terminal, you're good :)
Run npm -v
should print 8.15.0 or higher.

Run node -v
should print v18.7.0 or higher.

I say "or higher" because it depends when you run the installer. By that time, npm and NodeJS might have upgraded to higher versions. Just in case you're wondering :)
Install Truffle (your dev kit)
Truffle is a set of tools written in NodeJS, which help you with deploying locally, running tests, deploying to testnet & mainnet and much more. For more infos on what it has to offer check this out: https://trufflesuite.com/truffle/
Run npm install truffle -g
To check if it was installed fine, run truffle version
which should return something like this:

Install Ganache (your local blockchain)
Ganache is basically a blockchain that runs on your local machine. It mines blocks, it comes with 10 Ethereum addresses, which each get pre-filled with as much ETH as you want when you start it up.
Now you can tell Truffle to deploy to your local Blockchain (Ganache).
Once deployed, Truffle can run the tests you are written on those Smart Contracts - like a pro developer should, you have tests right :)
Ok let's install it.
We know that Ganache also offers an App you can install on your machine, which comes with a nice user interface to turn set up and manage the locally running blockchain. While that might sound appealing the app is a horror! It's consumes a ton of CPU which will bring your machine to its knees. Not sure what they messed up with that app but we don't recommend using it.
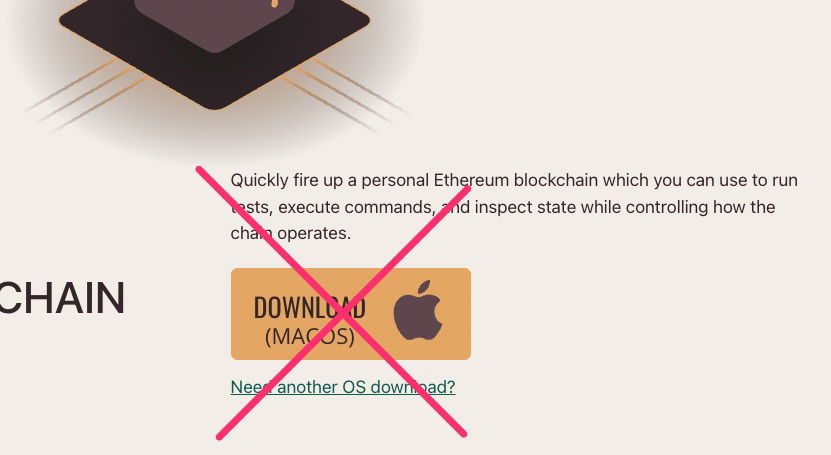
The long install guide is here: https://github.com/trufflesuite/ganache.
The short one is, run npm install ganache --global
.
Check if it has been properly installed by running ganache-cli --version
, which should print something like this:

Run your local Blockchain
Now that you have everything installed you can run your local blockchain for the first time 🥳
Run ganache-cli -e 1000000
to start your Ethereum compatible blockchain which comes with 10 addresses each owning 1,000,000 ETH 😱
Expect to see sth. like this:
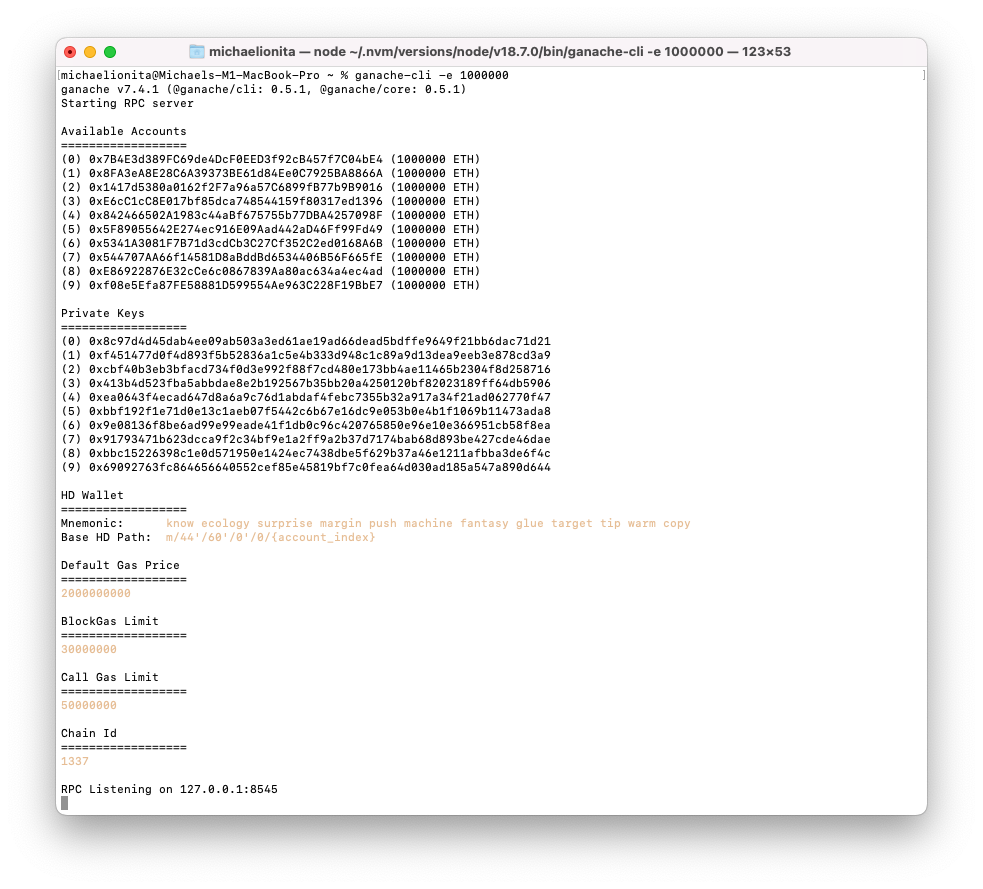
Now let's play around a bit with this.
Your Ganache Blockchain is running in that terminal window you executed the command in. Leave it open and don't touch it anymore!
Open a new terminal window and run truffle console
. You will then see sth. like this:

While you are inside the Truffle console you are in the proper environment to run truffle commands, which help you send and receive data to and from the blockchain.
Now run accounts
and you'll see all Ethereum addresses that you can use on your blockchain. Remember, each of these addresses as 1 million ETH at their disposal. Who wouldn't want that?!
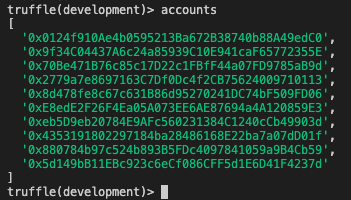
The first address (accounts[0]) from that list is used to deploy your Smart Contacts. Meaning, it's the OWNER and its private key will be used to deploy.That first address is SPECIAL. Don't treat it as a normal user wallet. It's the owner of all contacts you will deploy.
Will mention this a few times in this post because it's important :)
Do you notice that the addresses in this screenshot are different than in the one above?
That's because every time you re-start your ganache, aka. every time you run ganache-cli -e 1000000
you get a completely fresh blockchain with new addresses. That is actually a good thing, which keeps you from hardcoding addresses in your code 🤪
Run accounts[0]
and it will simply print you only the first address, because accounts is an array.

This will come in handy when we code later on as you can simply use accounts[0-9] to automate your testing for example. While accounts[0]
is the owner/admin/special one, which you should also treat this way aka. only use it for admin operations not as a normal user wallet when you run tests.
Install VS Code
You'll need some IDE to write your code in (aka. a text editor that is smart and helps you along the way).
We use VS Code because it's very well equipped with plugins for coding in Solidity.
First, install VS Code by download it from their website: https://code.visualstudio.com/
.
Then install these two extensions
:
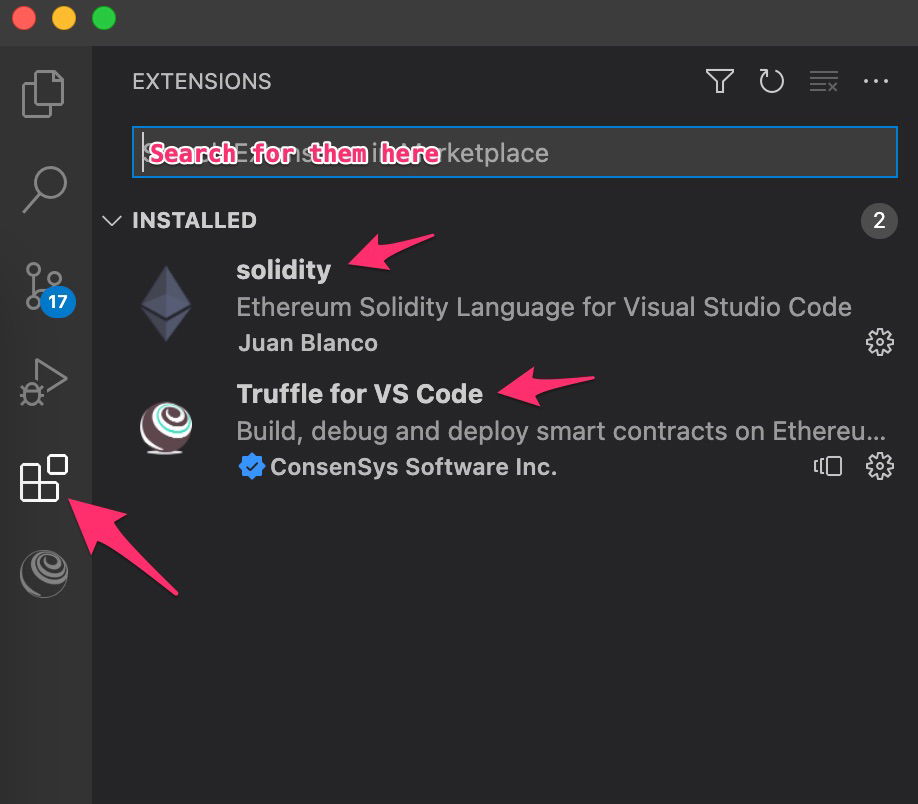
Code your first Smart Contract
Alright, here goes nothing 😋
The time has come.
Almost there.
Any minute now.
Ok seriously, all you need to do now is to download a sample truffle project.
For that, create a folder
where your project will be stored. Any folder works.
I use ~/Programming/PROJECT_NAME. But you can use whatever location you want.
The ~/ stands for the users "home folder" btw.
Go to your Terminal and open the folder there.
Run cd FOLDER_PATH
.

Once you are in that folder you can run truffle unbox metacoin
to download and unpack a sample Solidity project into that folder.
Easy stuff.
If you get stuck anywhere, make sure to join our Discord and ask your questions there. We are here for you.
Link below 👇🏼
https://discord.com/invite/J32drKNJtK
Inside the folder you will now find these files:
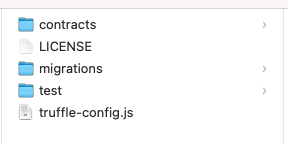
Inside contracts you'll find all smart contracts needed for this project (you can always add your own and delete the ones you don't need from that folder).
Inside migrations there is code for deploying the contracts. It's called "migrations" because not all contacts need to be deployed, sometimes you need to change some that are already deployed or you need to upgrade a contact (but that is for a more advanced post). Also here, you can add your own migrations. We will show you how in this blogpost.
Open project in VS Code
Now that you have the project downloaded, open it in VS Code, which will make your life easier.
Open VS Code, and click on Open Folder
and pick the folder you have created before (the folder contains contracts, migrations etc.).
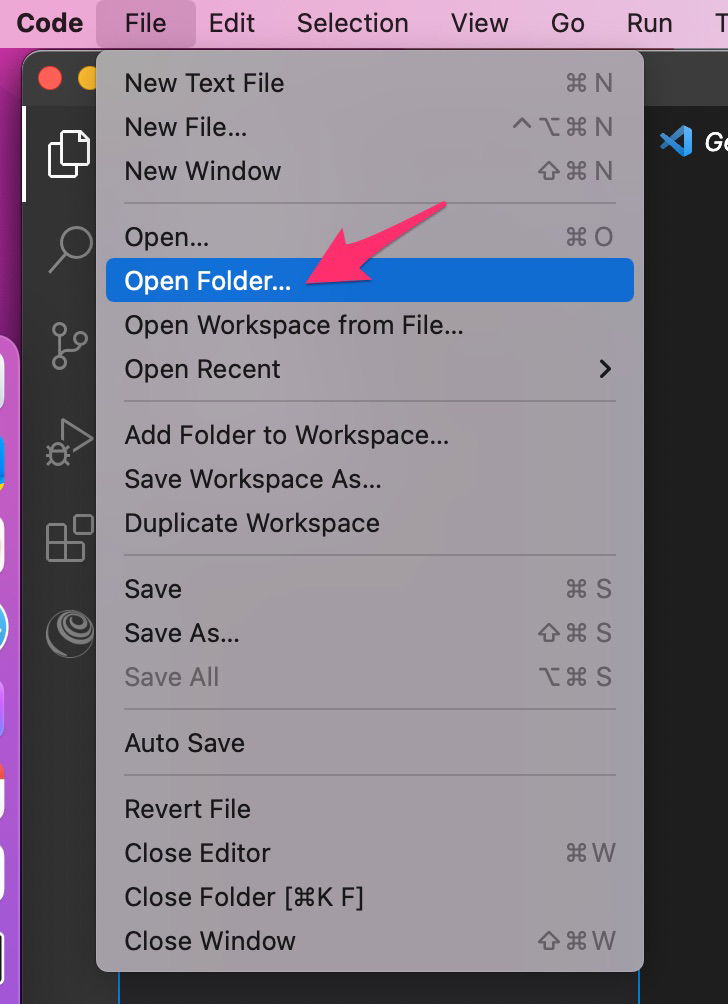
Once your project is open in VS Code it will look something like this:
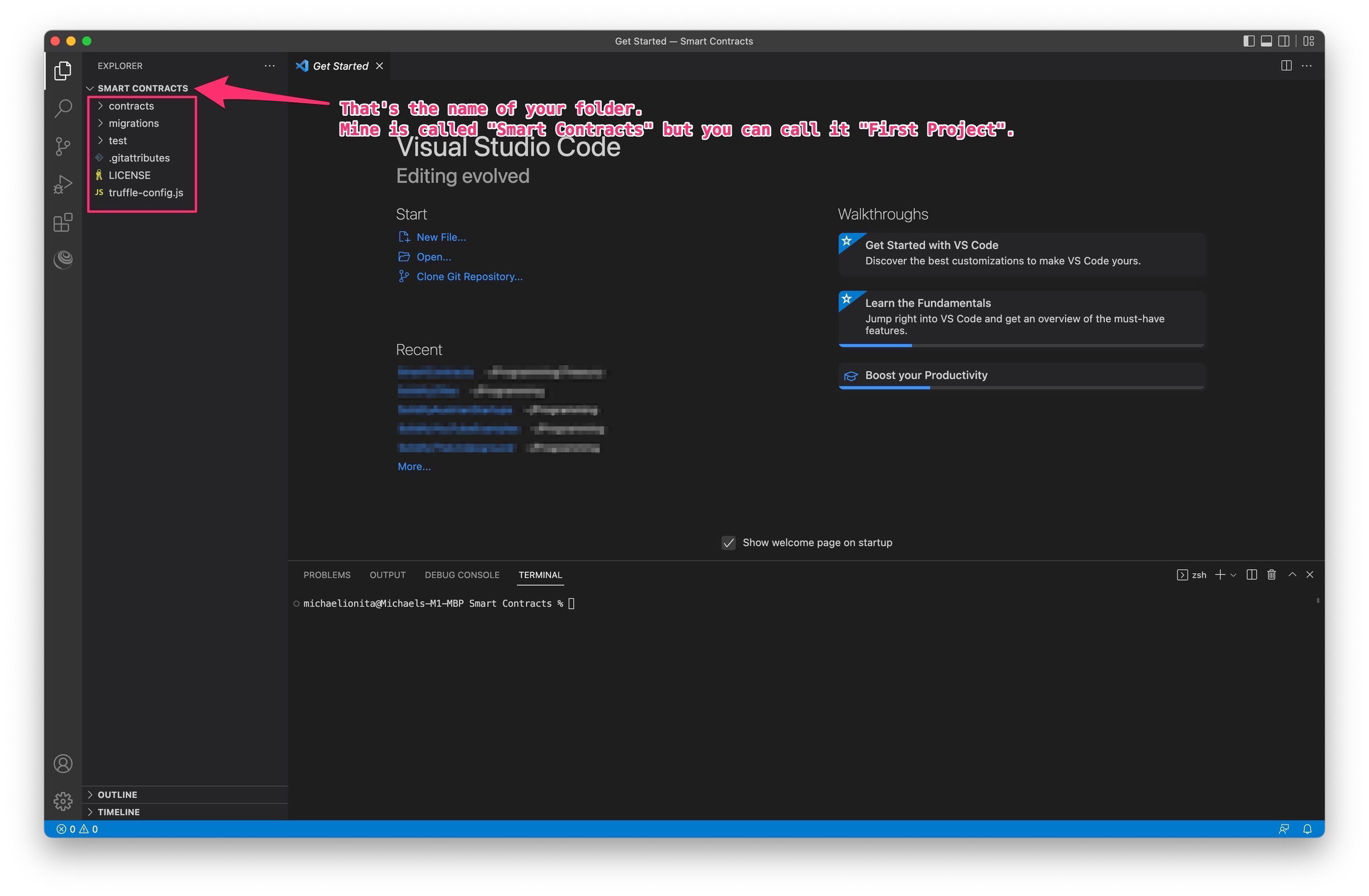
Now, open the Terminal in VS Code
You can now open the Terminal in VS Code from the menu.
This is useful because the terminal window will automatically be pointing to the project folder, which saves you the switch to the right folder.
Such small conveniences make a difference in daily coding and save us time :)
Click on Terminal
in the menu and click on New Terminal
:
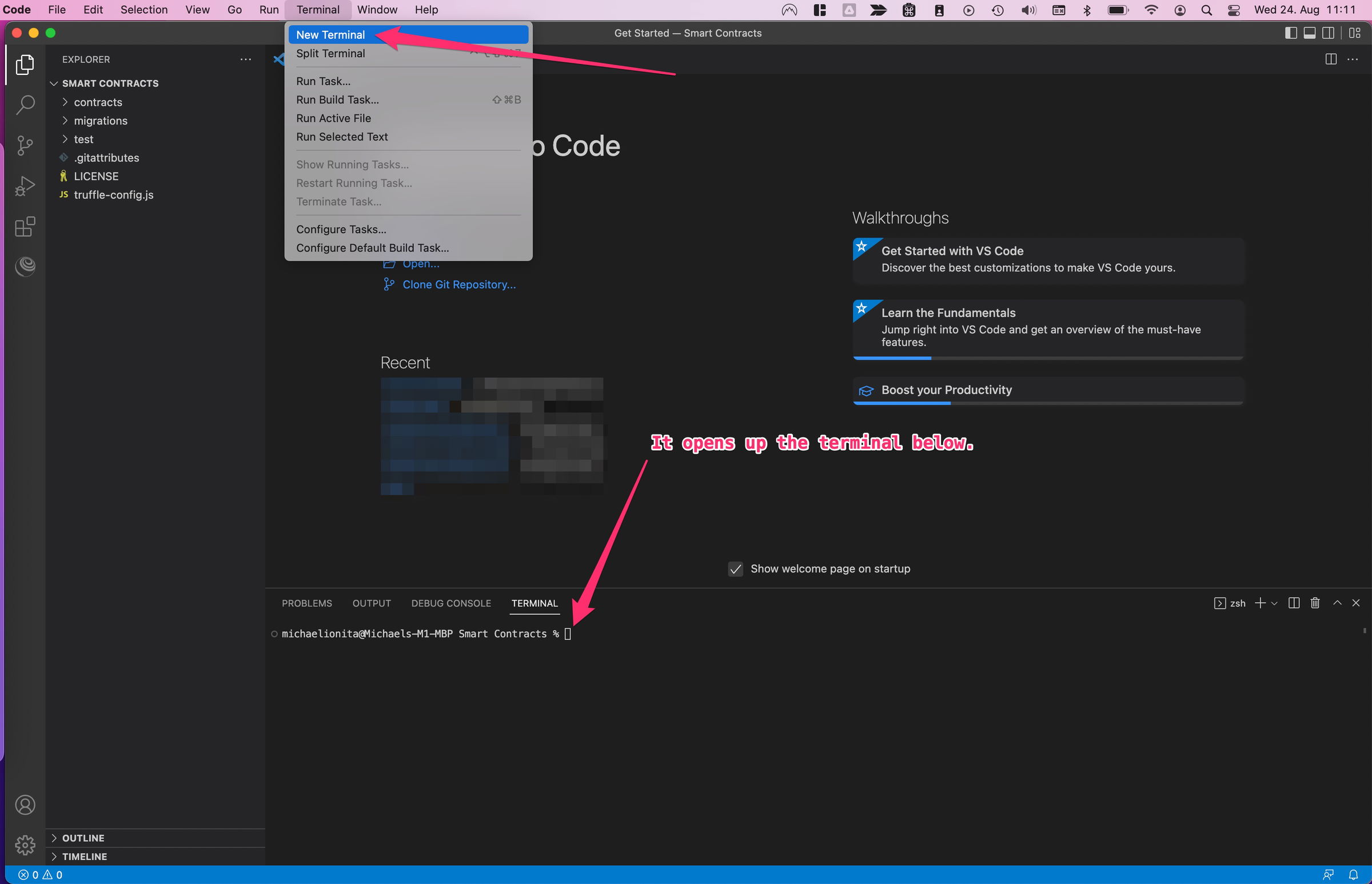
Deploy the project
First we need to edit the truffle-config.js file
to tell Truffle where Ganache is running.
Remember, Ganache is your locally running blockchain and it runs on a port on your localhost. Localhost has a fixed IP address, which is 127.0.0.1.
Uncomment
these lines in the truffle-config.js
file:
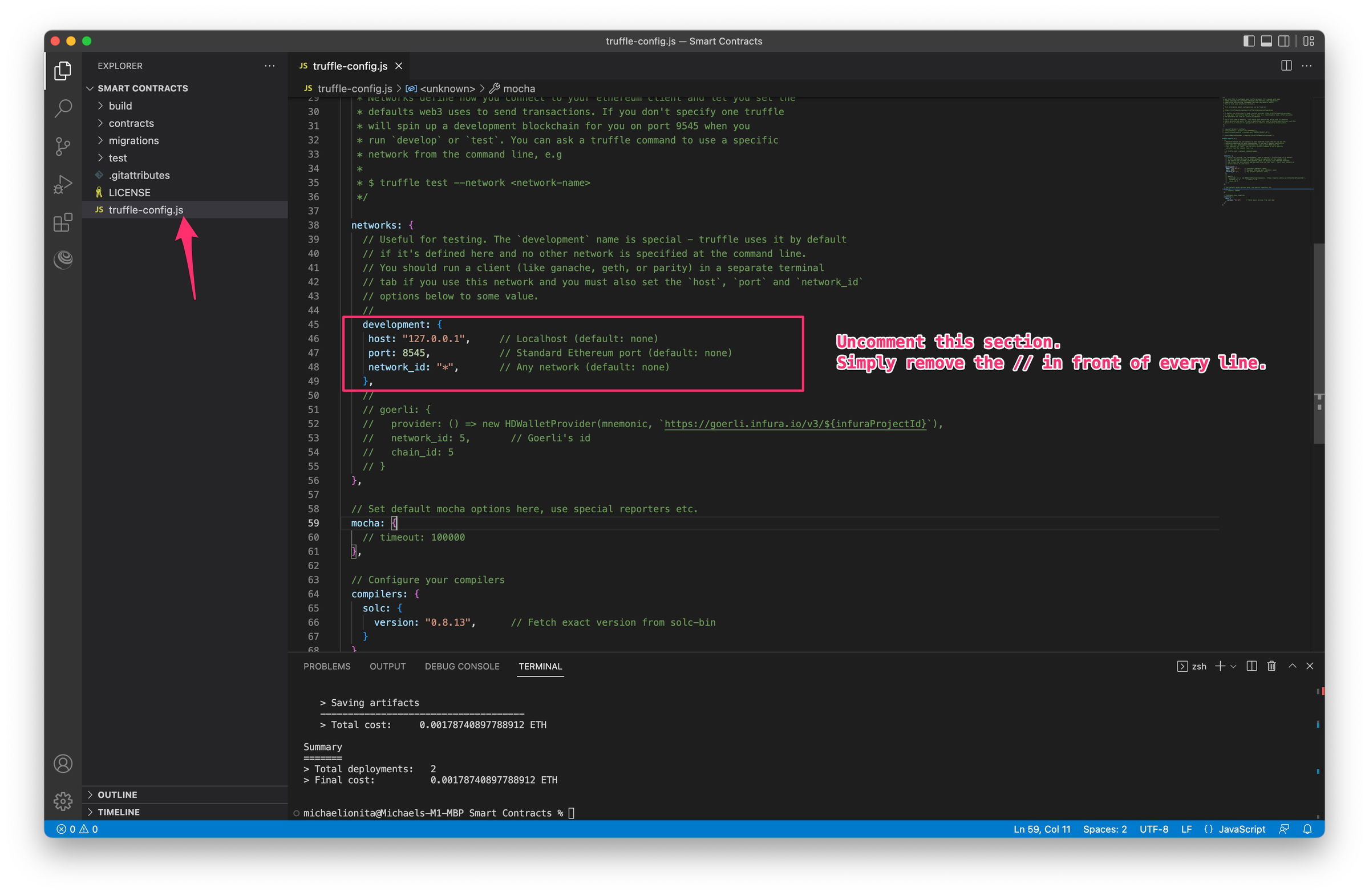
Now, in the VS Code Terminal, run truffle migrate --reset
in the VS Code terminal.
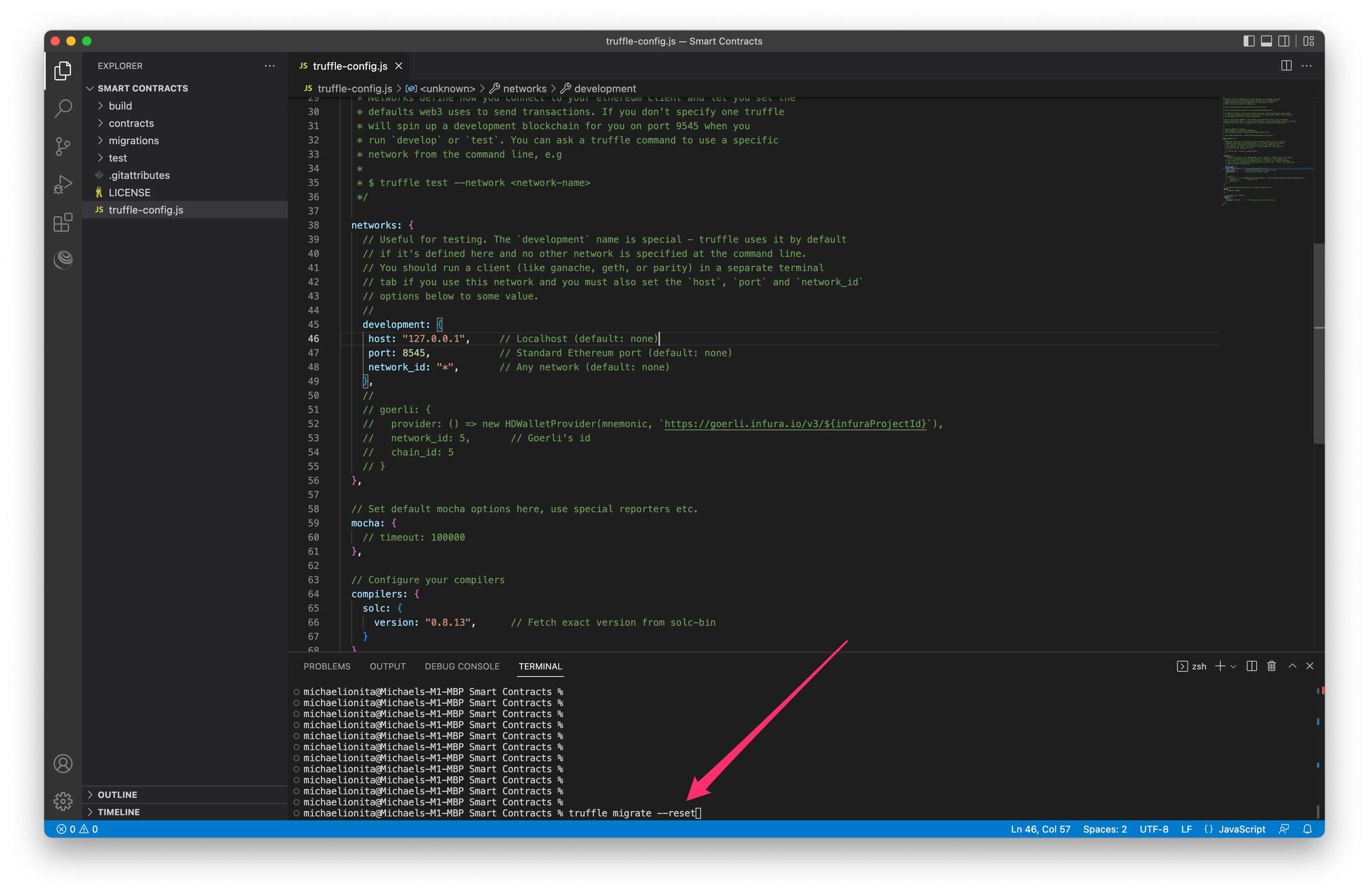
If you get an error message most likely the other Terminal window where Ganache was running has been closed by now (depending if you do this tutorial in one go or on multiple days).
Simply make sure to have Ganache running (we explained how above). I usually run Ganache outside of VS Code (in a separate Terminal window) simply because I need the VS Code Terminal to be available to me at all times and running Ganache basically blocks the Terminal window for the Ganache process.
If you can see the below output your smart contract is now running 🥳
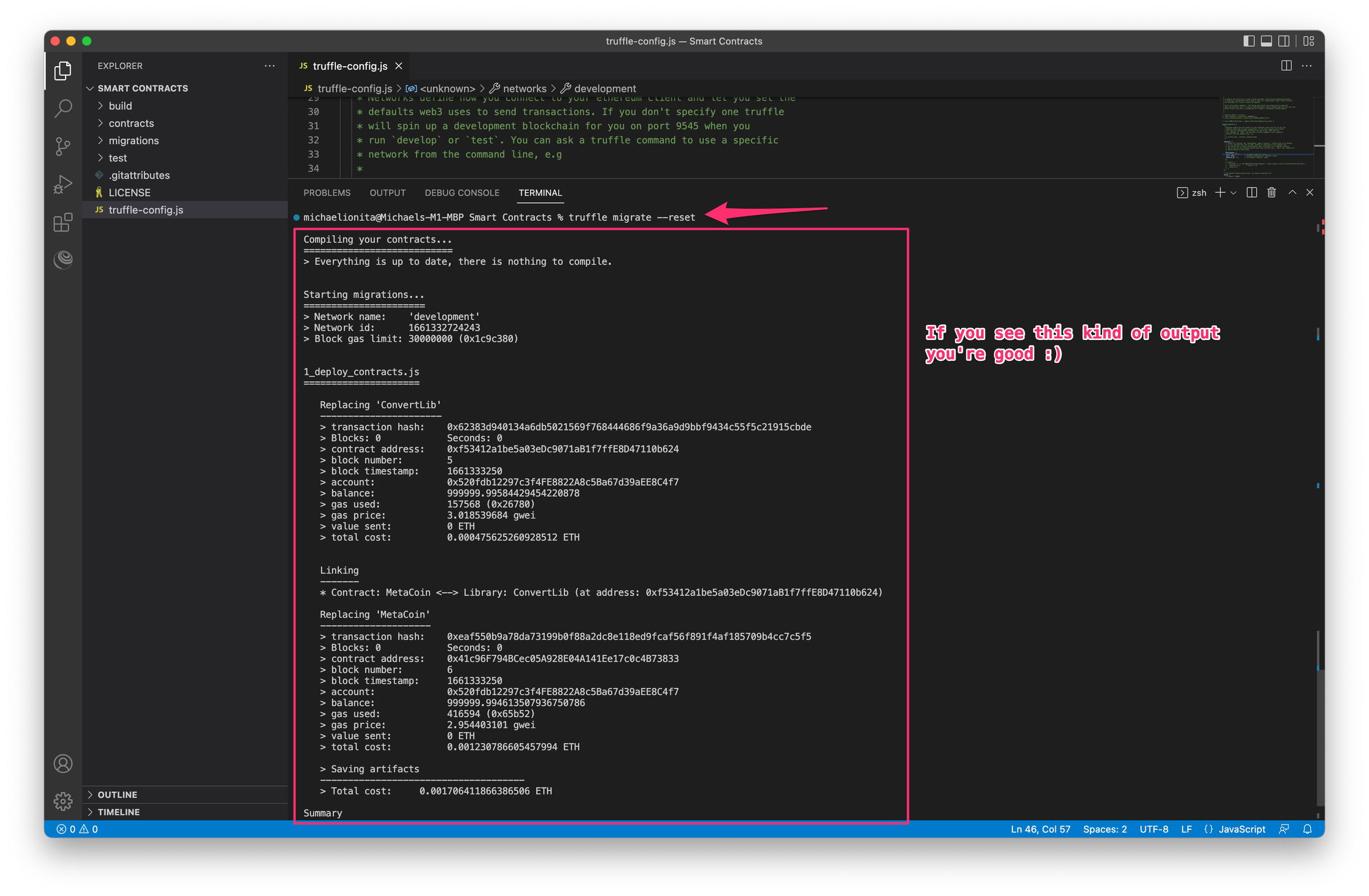
BOOM! Nice.
You have deployed your first smart contract onto your local blockchain 💪🏼
Interact with your Smart Contract
Now let's call a function from the smart contract to see what it returns.
In your VS Code terminal run truffle console
.

You should see truffle(development)> in the next line in your console (like before).

If you are already in the truffle console then you don't need to run truffle console of course.
Now we need to tell Truffle, which smart contract we want to talk to.
For that, run this command const MetaCoinContract = await MetaCoin.deployed()
.
This tells Truffle to create an instance of the deployed smart contract and store it into a variable called MetaCoinContract.
Now let's call the smart contract itself (moment of truth).
Run MetaCoinContract.getBalance(accounts[1])
.
You should see something like this:
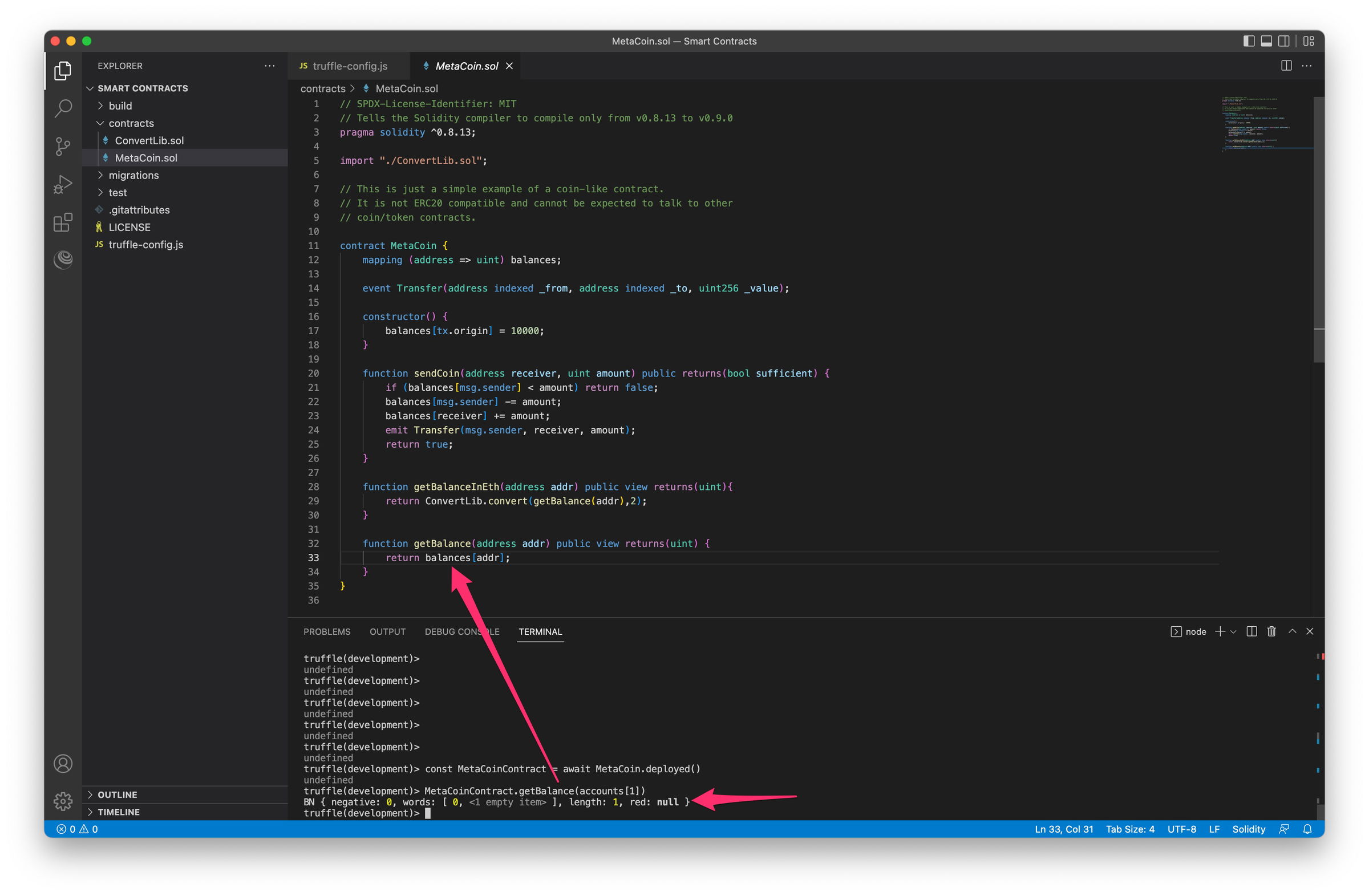
The BN { negative: 0, words: [ 0, <1 empty item> ], length: 1, red: null } is basically a variable type called Big Number. It's just a way for the blockchain (and other programming languages) to work with really big numbers. I'm talking about numbers like this 1000000000000000000000000 for example.
Why do we need such numbers?
Because on the blockchain there is no comma or floating point.
Why? Because floating point calculations are error prone - they can lead to different results with many numbers after the comma. But let's not go into this topic too much as it's huge :)
Just remember that 1 ETH = 1000000000000000000.
Those are 18 zeros.
So if you want 0.1 ETH you simply remove one zero.
0.1 ETH = 100000000000000000.
Those are 17 zeros.
And if you want 10 ETH you add one zero (easy).
10 ETH = 10000000000000000000.
Those are 19 zeros.
This way you can represent any number without commas while still being able to have commas. Crazy right :) In the finance sector this is is common practice because calculating with commas/floating point numbers, is not accurate as there can potentially be infinite numbers after the comma - not a great thing in computers, which can't calculate with infinite numbers, nobody really can.
In Solidity, there are no commas!
You can print a Big Number to a String (just text) when you wrap the previous command like so: (await MetaCoinContract.getBalance(accounts[1])).toString()
The .toString() part prints the number as a text. You can't use that for calculations anymore but you can at least see it with your eyes easier (can help with debugging for example).
When reading and writing from and to Smart Contracts, we recommend to do ALL your calculations with Big Numbers to avoid conversion bugs.
More on that later.
As you can see the user has 0 funds in that smart contract. Boring.

Let's give accounts[1] some funds.
Run MetaCoinContract.sendCoin(accounts[1], "100")
.
You should now see something like this:
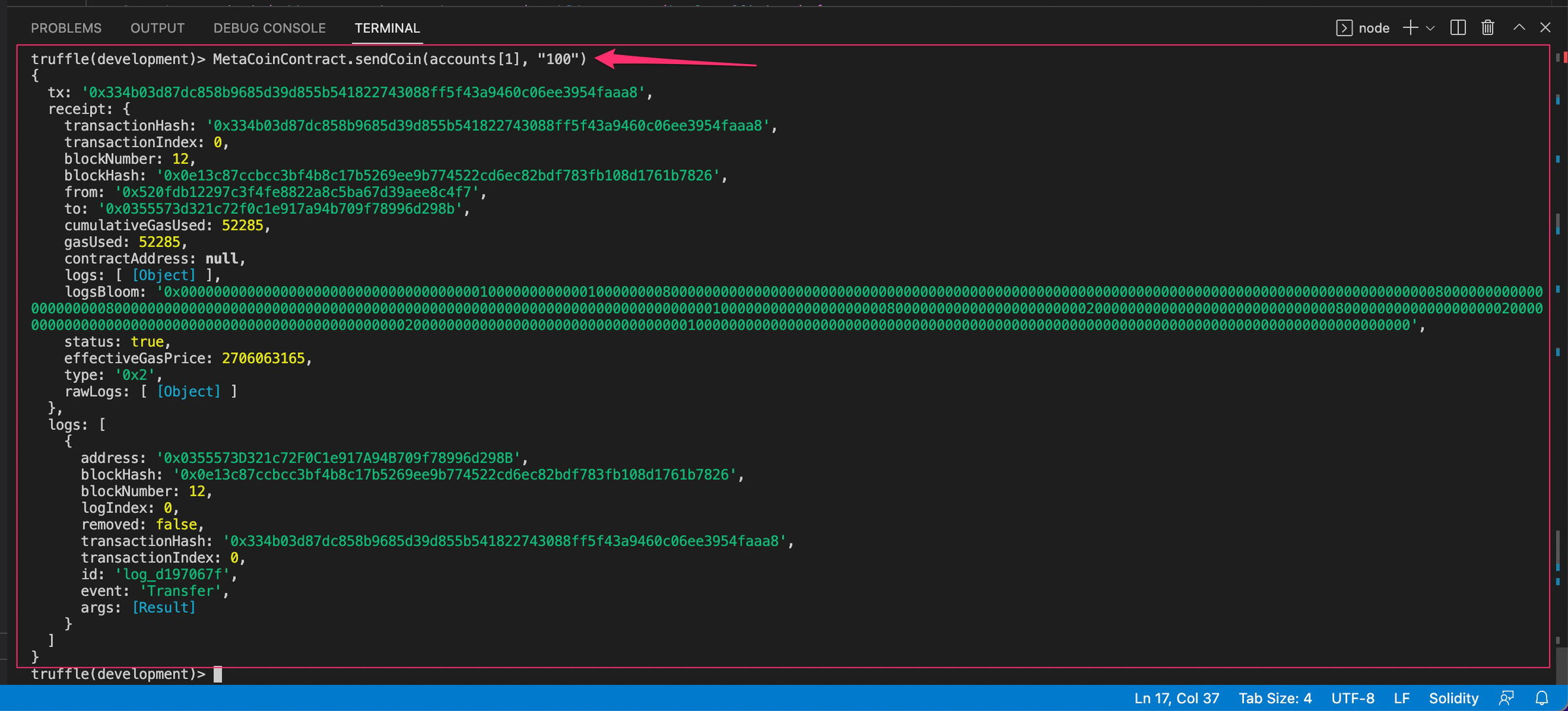
Cool!
Now when you run (await MetaCoinContract.getBalance(accounts[1])).toString()
again, you will see that this account has some funds in there.
Btw. you can simply press the "arrow up" on your keyboard to see previous commands you have executed.
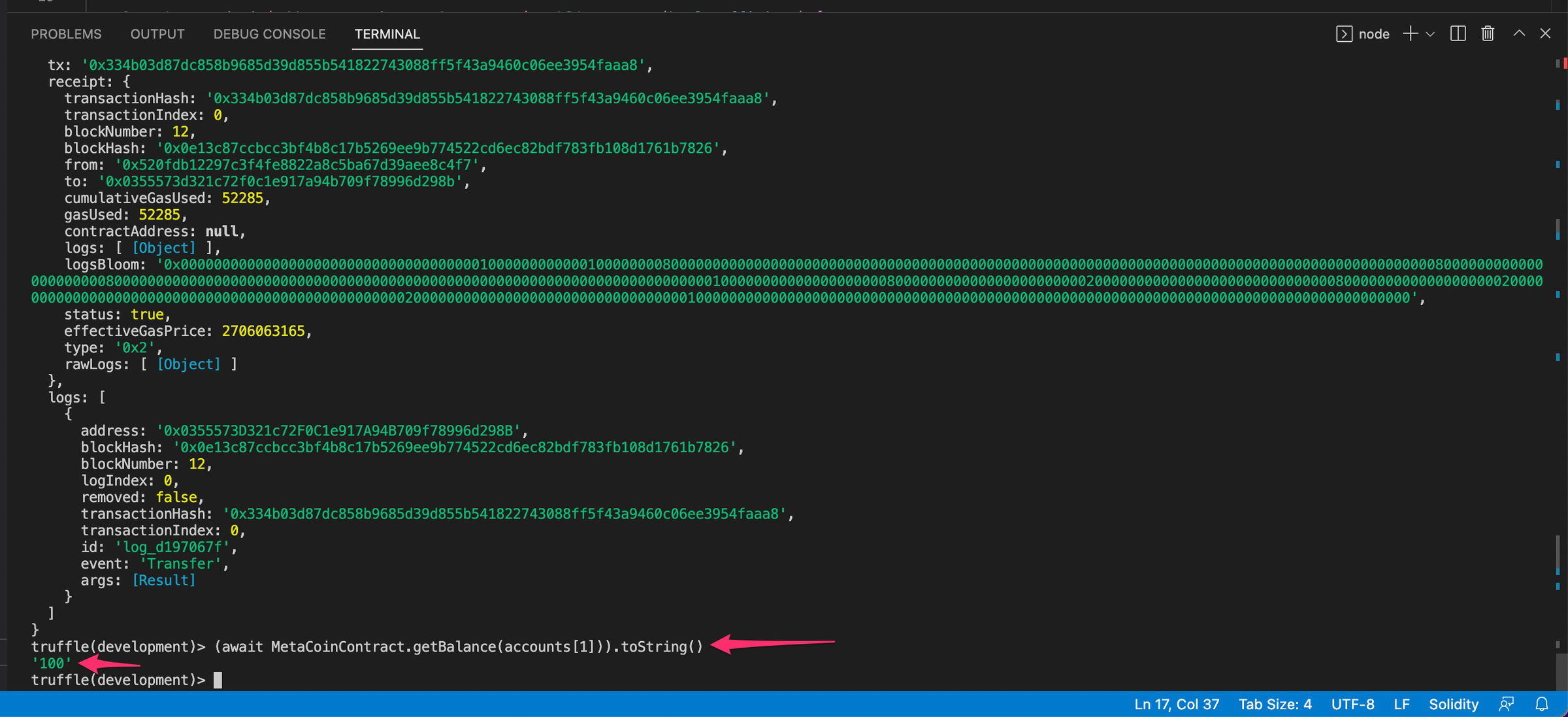
Nice, you have sent funds from accounts[0] to accounts[1].
But, you have to understand one more thing.
When you ran MetaCoinContract.sendCoin(accounts[1], "100") truffle automatically assumed that you are sending this transaction from accounts[0] as this is the default behaviour on your local development environment.
So what it ACTUALLY executed was:
MetaCoinContract.sendCoin(accounts[1], "100", {from: accounts[0]})
You see it added the {from: accounts[0]} to the command automatically.
This is cool because now you can use that to your advantage.
You can now run this command for accounts[1] as well and send funds from that account to some other account.
Run MetaCoinContract.sendCoin(accounts[0], "100", {from: accounts[1]})
With this command account 1 will simply send 100 units back to account 0.
Of course you can use any account from 0 - 9 to play around.
On the actual blockchain (testnet or mainnet) there is NO WAY you can run transactions from a wallet you don't own.
You can do this locally because you own ALL 10 test accounts on your machine (accounts[0-9]).
Is this smart contract secure?
Kind of.
Look at this code and read the annotation inside to understand what the code does:
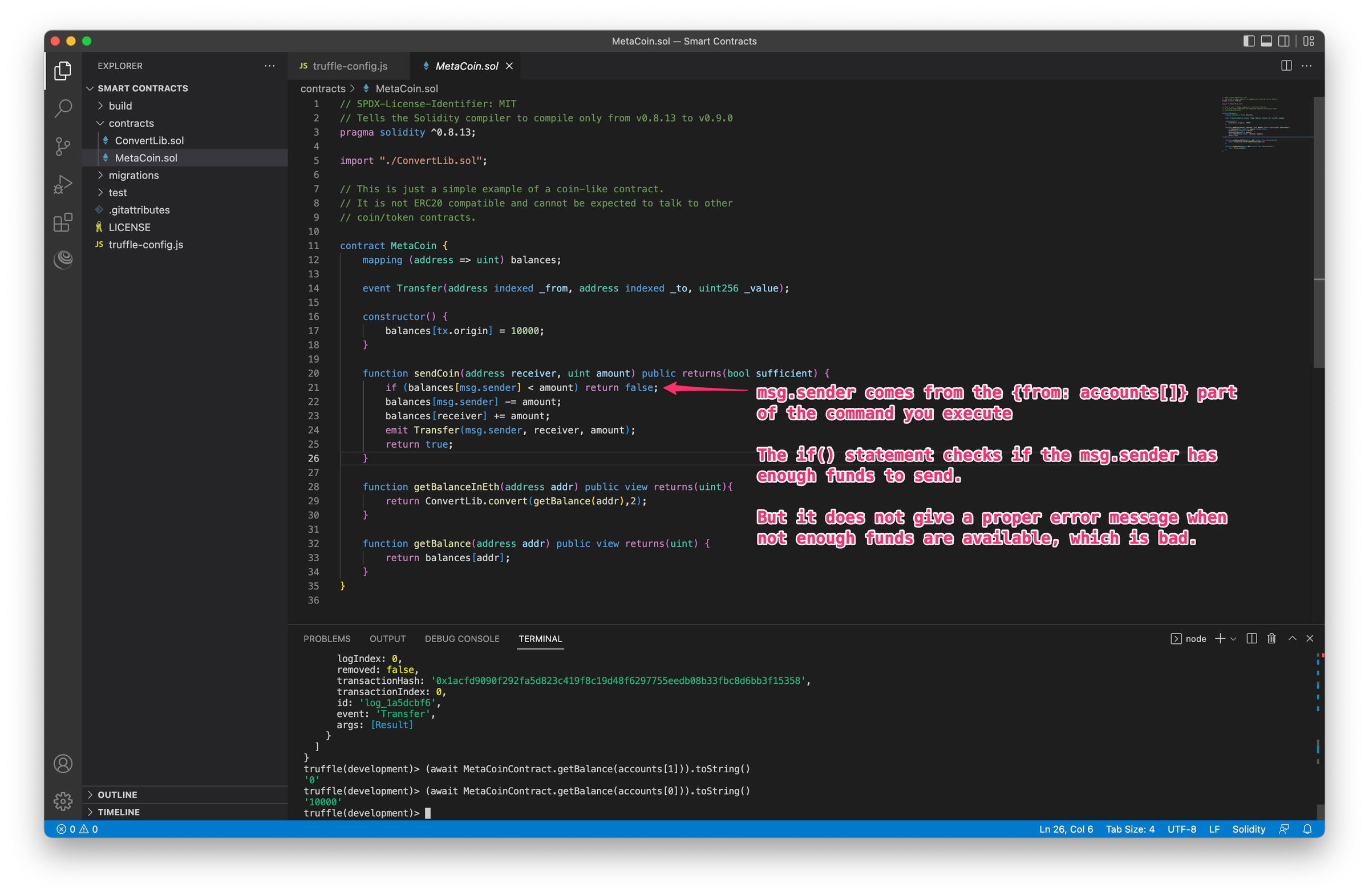
Next steps will be to edit & improve the Solidity Smart Contract and deploy it onto Testnet where others can also interact with it.
This blogpost will be updated very soon. So make sure to reload the page daily.
Meanwhile you can join our Discord if you have questions: https://discord.com/invite/J32drKNJtK